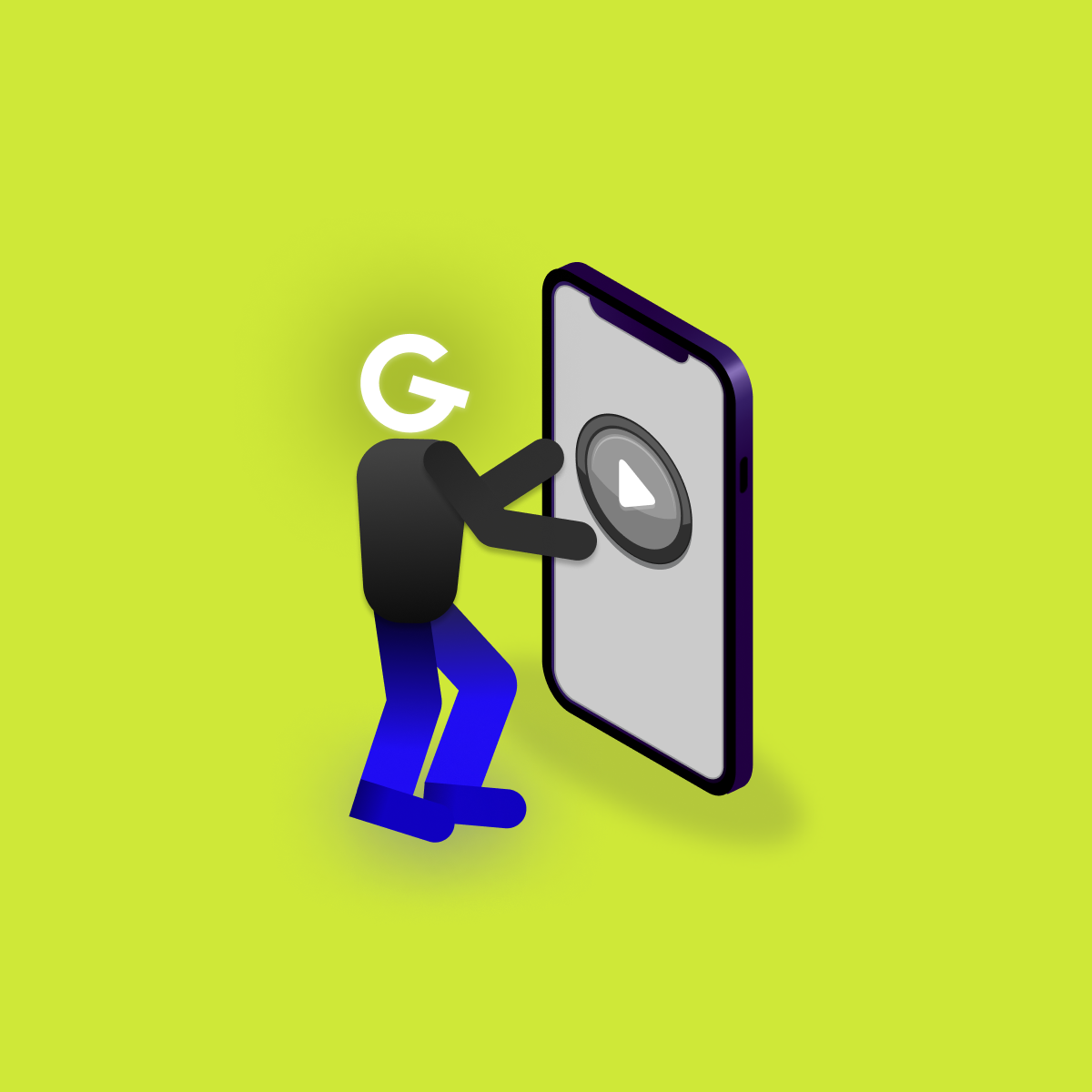
Embedded games in mobile apps: Make your app more attractive

Backdrop component is composed of 2 surfaces: back layer and front layer. Purpose of back layer is to display context. Part of the it is appBar. Purpose of front layer is to show content based of context from back layer. Backdrop is implemented in Jetpack Compose as BackdropScaffold composable.
Don't forget to check out previous articles in the Jetpack compose basics series. We have already covered Scaffold, Snackbar, Showing Image, Text Input, Modal Bottom Sheet and Composition local.
BackdropScaffold, as usual in Jetpack Compose, has many parameters for customization, three of them are mandatory
@Composable
fun BackdropScaffold(
appBar: @Composable () -> Unit,
backLayerContent: @Composable () -> Unit,
frontLayerContent: @Composable () -> Unit,
modifier: Modifier = Modifier,
scaffoldState: BackdropScaffoldState = rememberBackdropScaffoldState(Concealed),
gesturesEnabled: Boolean = true,
peekHeight: Dp = BackdropScaffoldDefaults.PeekHeight,
headerHeight: Dp = BackdropScaffoldDefaults.HeaderHeight,
persistentAppBar: Boolean = true,
stickyFrontLayer: Boolean = true,
backLayerBackgroundColor: Color = MaterialTheme.colors.primary,
backLayerContentColor: Color = contentColorFor(backLayerBackgroundColor),
frontLayerShape: Shape = BackdropScaffoldDefaults.frontLayerShape,
frontLayerElevation: Dp = BackdropScaffoldDefaults.FrontLayerElevation,
frontLayerBackgroundColor: Color = MaterialTheme.colors.surface,
frontLayerContentColor: Color = contentColorFor(frontLayerBackgroundColor),
frontLayerScrimColor: Color = BackdropScaffoldDefaults.frontLayerScrimColor,
snackbarHost: @Composable (SnackbarHostState) -> Unit = { SnackbarHost(it) }
)
Other parameters are optional:
Most of them is easy to understand, some of them are little harder. Examples will be demonstrated on BackdropScaffold looking like this. It has appBar with navigation arrow and title. Back layer with 2 text fields and front layer with list of cats.
Height of visible part of back layer when it is concealed. In this example peekHeight is set to 100dp, in simpler words, when back layer is concealed 100dp of it will be visible.
peekHeight = 100.dp
If front layer should stick to height of back layer. Default value is true so the top of front layer is sticked to bottom of back layer. When false is set and headerHeight is left with default value (56dp), then only 56dp of front layer will be visible. In example headerHeight is not modified and stickyFrontLayer is set to false.
stickyFrontLayer = false
Minimum height of front layer when it is inactive. In this example, headerHeight is set to 100dp. stickyFrontLayer need to be set to false if we want that small height of front layer otherwise front layer will stick to height of back layer.
headerHeight = 100.dp,
stickyFrontLayer = false
If the app bar should be shown when back layer is revealed. In this example, initial state of back layer is revealed and toolbar is not visible. With the gesture, state transitions to concealed which shows app bar.
persistentAppBar = false
This is example of backdrop without any modifications. Starting from revealed state of back layer with persistent app bar and front layer sticking to back layer.
Looking for future in Android development? We're looking for new teammates!