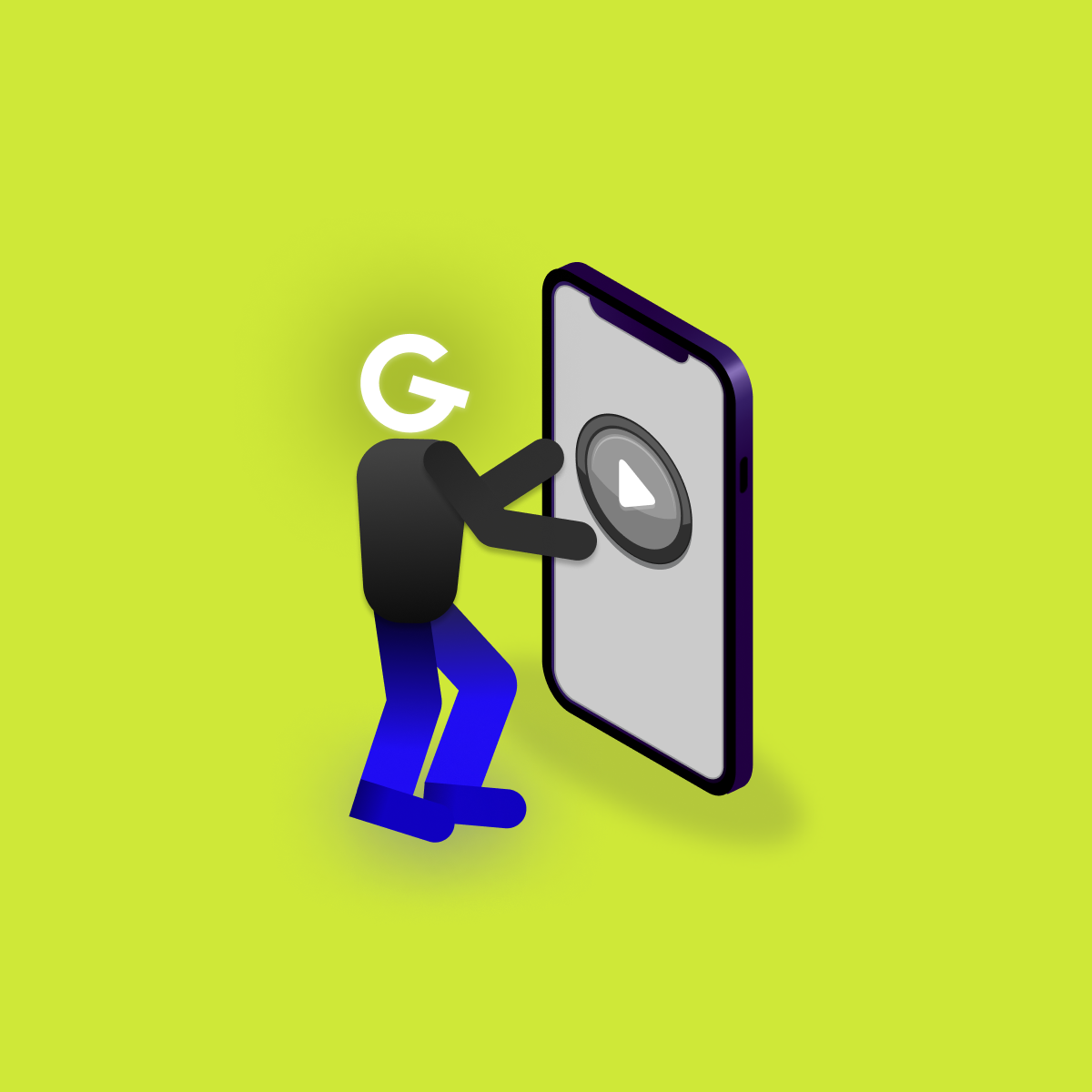
Embedded games in mobile apps: Make your app more attractive

4. Oct 2021
AndroidImages and Icons are crucial part of your app’s UI used for visually expressing actions. Also, they help to make an overall impression of your app, make an app unique. How to work with them in Jetpack Compose? Let’s find out.
In Jetpack Compose there are 3 types of Image composable out of the box. First takes bitmap as parameter, second takes vector and the last takes painter. These functions have many parameters in common.
@Composable
fun Image(
//bitmap or vector or painter
contentDescription: String?,
modifier: Modifier = Modifier,
alignment: Alignment = Alignment.Center,
contentScale: ContentScale = ContentScale.Fit,
alpha: Float = DefaultAlpha,
colorFilter: ColorFilter? = null
)
This snippet of code loads and shows given bitmap.
val bitmap = getBitmapFromYourSource()
Image(
bitmap = bitmap,
contentDescription = "Cat"
)
This snippet of code loads and shows given vector, e.g. icon.
This is the most interesting part. Both bitmap and vector uses this type of image composable on the background. There are many predefined painters e.g. ColorPainter which draws defined color in bounds.
Image(
painter = ColorPainter(Color.Yellow),
contentDescription = "Yellow rectangle"
)
You probably don't have all images used in application saved locally. If your application works with API which sends you URL of image, you will need to load it from internet. That is where you should use any of available libraries.
Shortly before release of first stable version of Jetpack Compose, developers of Coil image loader announced their library fully works with Compose. Before this event, the best solution was to use Accompanist coil image loader.
Coil has many great features and on the background implements painter API too.
What if we want this type of "layout" in the applications where we obtain images as url? It is really simple, remember the painter and throw it as parameter into Image composable.
Row(
modifier = Modifier.padding(16.dp),
horizontalArrangement = Arrangement.spacedBy(16.dp)
) {
//Important image loading part
//Remembering of painter
val painter = rememberImagePainter(data = cat.photoUrl)
Image(
modifier = Modifier
.size(100.dp)
.clip(RoundedCornerShape(16.dp)),
//Use painter in Image composable
painter = painter,
contentDescription = "Cat"
)
//Rest of compose code
}
Earlier, we mentioned that Coil has many great features e.g. scaling, transformations, memory caching, bitmap polling..., basically everything you will need. To use them is simple, call wanted feature inside builder. These we use most of the time.
val painter = rememberImagePainter(
data = url,
builder = {
crossfade(true) //Crossfade animation between images
placeholder(R.drawable.ic_image_loading) //Used while loading
fallback(R.drawable.ic_empty) //Used if data is null
error(R.drawable.ic_empty) //Used when loading returns with error
}
)
Voilà, images are now loading almost without effort.