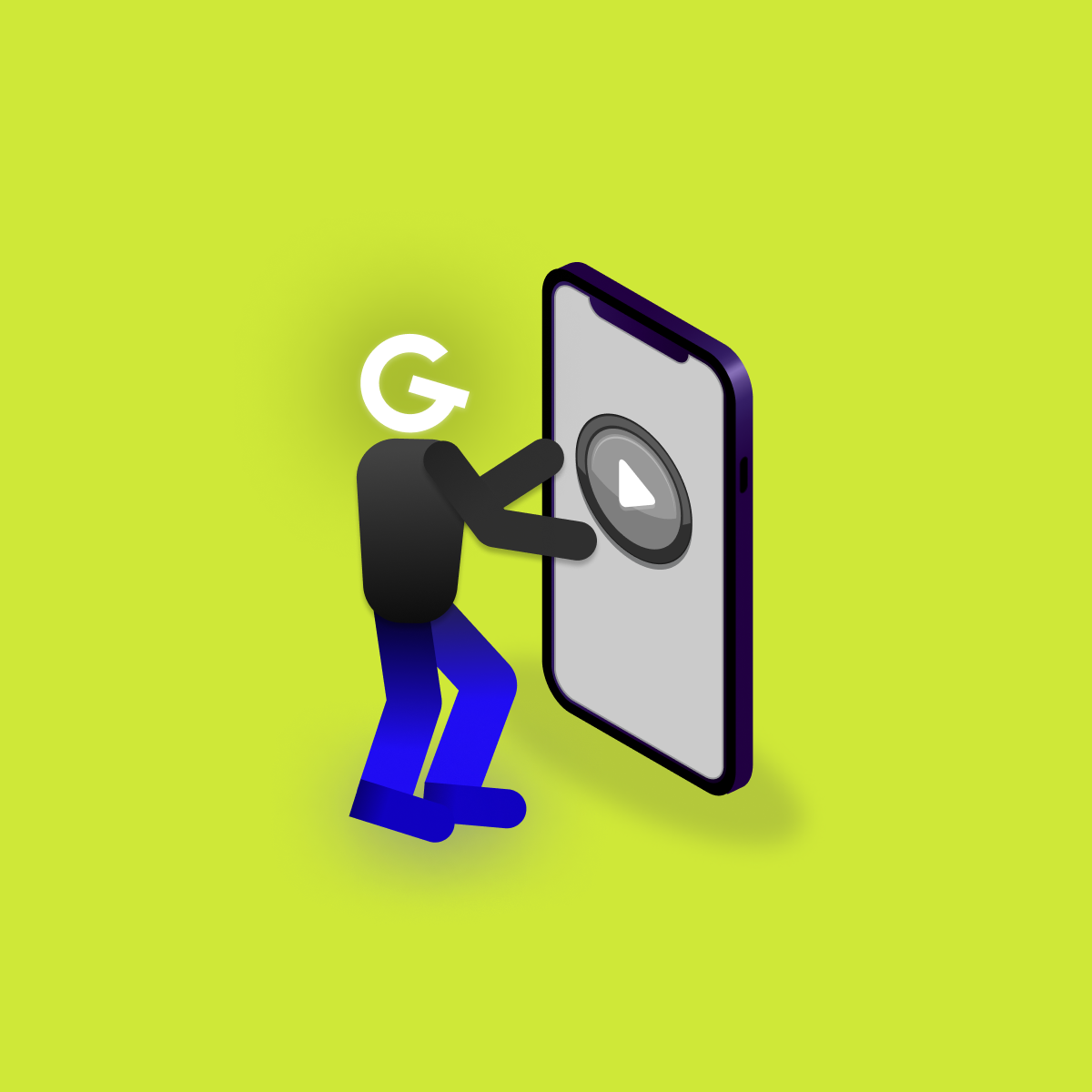
Embedded games in mobile apps: Make your app more attractive

13. Dec 2021
AndroidBottom Sheet is component used as supplementary surface anchored to bottom of screen. This article will be about exact type of Bottom Sheet - Modal Bottom Sheet and how to use it in Jetpack Compose.
It's an alternative to menus or dialogs, it has to be dismissed (either by swipe or by some other user interaction) to interact with underlaying content of screen. In Jetpack Compose, bottom sheet is implemented via ModalBottomSheet composable.
ModalBottomSheet has 3 possible state defined through sheetState parameter of type ModalBottomSheetValue. Possible values are Hidden, Expanded or HalfExpanded. Default value in sheet state is Hidden, own state can be remembered like this
val sheetState = rememberModalBottomSheetState(initialValue = ModalBottomSheetValue.Hidden)
ModalBottomSheet, as usual in Jetpack Compose, has many parameters for customisation, two of them are mandatory.
fun ModalBottomSheetLayout(
sheetContent: @Composable ColumnScope.() -> Unit,
modifier: Modifier = Modifier,
sheetState: ModalBottomSheetState = rememberModalBottomSheetState(Hidden),
sheetShape: Shape = MaterialTheme.shapes.large,
sheetElevation: Dp = ModalBottomSheetDefaults.Elevation,
sheetBackgroundColor: Color = MaterialTheme.colors.surface,
sheetContentColor: Color = contentColorFor(sheetBackgroundColor),
scrimColor: Color = ModalBottomSheetDefaults.scrimColor,
content: @Composable () -> Unit
)
There are show() and hide() functions ready for animated transition of ModalBottomSheet which can be called on modalBottomSheetState. These are suspend functions, because animation run as coroutine, scope or LaunchedEffect is needed to use them.
val sheetState = rememberModalBottomSheetState(initialValue = ModalBottomSheetValue.Hidden)
Button(
onClick = {
scope.launch {
// Important part
sheetState.show()
}
},
content = { ... }
)
In some cases, HalfExpanded state is not desirable and luckily there is a way to disable it by returning false in confirmStateChange lambda.
val sheetState = rememberModalBottomSheetState(
initialValue = ModalBottomSheetValue.Hidden,
confirmStateChange = { it != ModalBottomSheetValue.HalfExpanded }
)