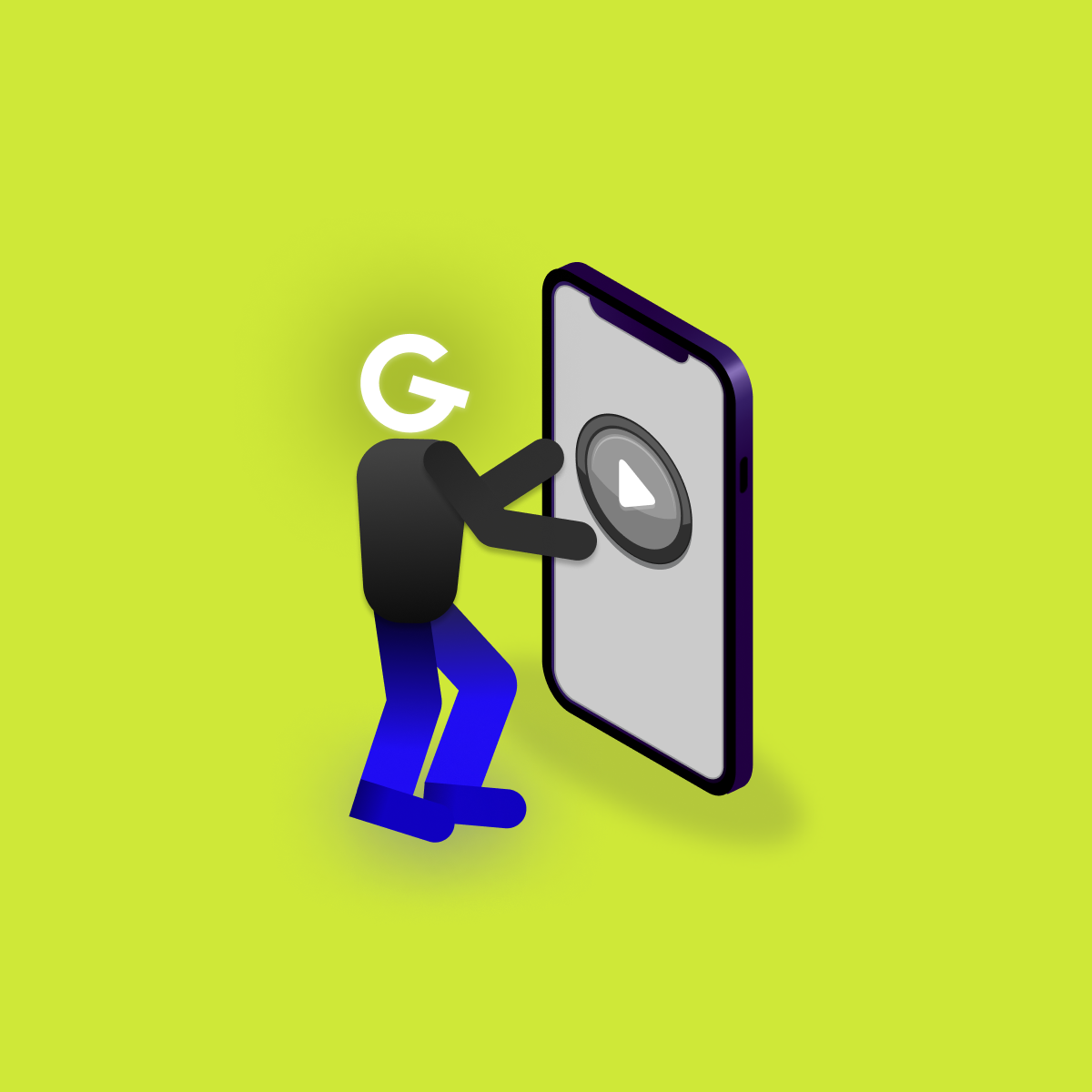
Embedded games in mobile apps: Make your app more attractive

2. Nov 2021
AndroidText field is UI component that allow users to enter textual information into a UI, typically in forms. Text fields in Material design come in two types: Filled text fields or Outlined text fields. Both provide the same functionality, so your choice should be based on style preferences. It is not recommended to mix them.
Both text field types consist of container (1), optional leading icon (2), label (3), input text (4), optional trailing icon (5), activation indicator (6) and optional helper text (7)
Compose provides OutlinedTextField composable to fulfill Material specification of outlined text field. Without further customisation OutlinedTextField composable looks like this
This composable has around 20 parameters but only 2 of them are mandatory.
@Composable
fun OutlinedTextField(
value: String,
onValueChange: (String) -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
readOnly: Boolean = false,
textStyle: TextStyle = LocalTextStyle.current,
label: @Composable (() -> Unit)? = null,
placeholder: @Composable (() -> Unit)? = null,
leadingIcon: @Composable (() -> Unit)? = null,
trailingIcon: @Composable (() -> Unit)? = null,
isError: Boolean = false,
visualTransformation: VisualTransformation = VisualTransformation.None,
keyboardOptions: KeyboardOptions = KeyboardOptions.Default,
keyboardActions: KeyboardActions = KeyboardActions.Default,
singleLine: Boolean = false,
maxLines: Int = Int.MAX_VALUE,
interactionSource: MutableInteractionSource = remember { MutableInteractionSource() },
shape: Shape = MaterialTheme.shapes.small,
colors: TextFieldColors = TextFieldDefaults.outlinedTextFieldColors()
)
Optional parameters which can customize text field are
label = { Text(text = "Label for text") }
placeholder = { Text(text = "Placeholder for text") }
leadingIcon = { Icon(imageVector = Icons.Default.Info, contentDescription = "") }
//OR
leadingIcon = { Text(text = "$") }
visualTransformation = PasswordVisualTransformation()
keyboardOptions = KeyboardOptions.Default.copy(
capitalization = KeyboardCapitalization.Words,
autoCorrect = false,
keyboardType = KeyboardType.Text,
imeAction = ImeAction.Search
)
keyboardActions = KeyboardActions(
onAny = {} // do when ANY of ime actions is emitted
)
//OR
keyboardActions = KeyboardActions(
onDone = {}, // do when SPECIFIED action is emitted
onSearch = {},
.
.
}
colors = TextFieldDefaults.outlinedTextFieldColors(
focusedBorderColor = Color.Yellow,
leadingIconColor = Color.Yellow
)
Compose provides TextField composable to fulfill Material specification of filled text field. Without further customization TextField composable looks like this
All parameters of TextField composable function are the same as for OutlinedTextField (except different shape and colors) and have the same usage.
TextField with enabled as false
TextField with label
TextField with leading icon
TextField in error state