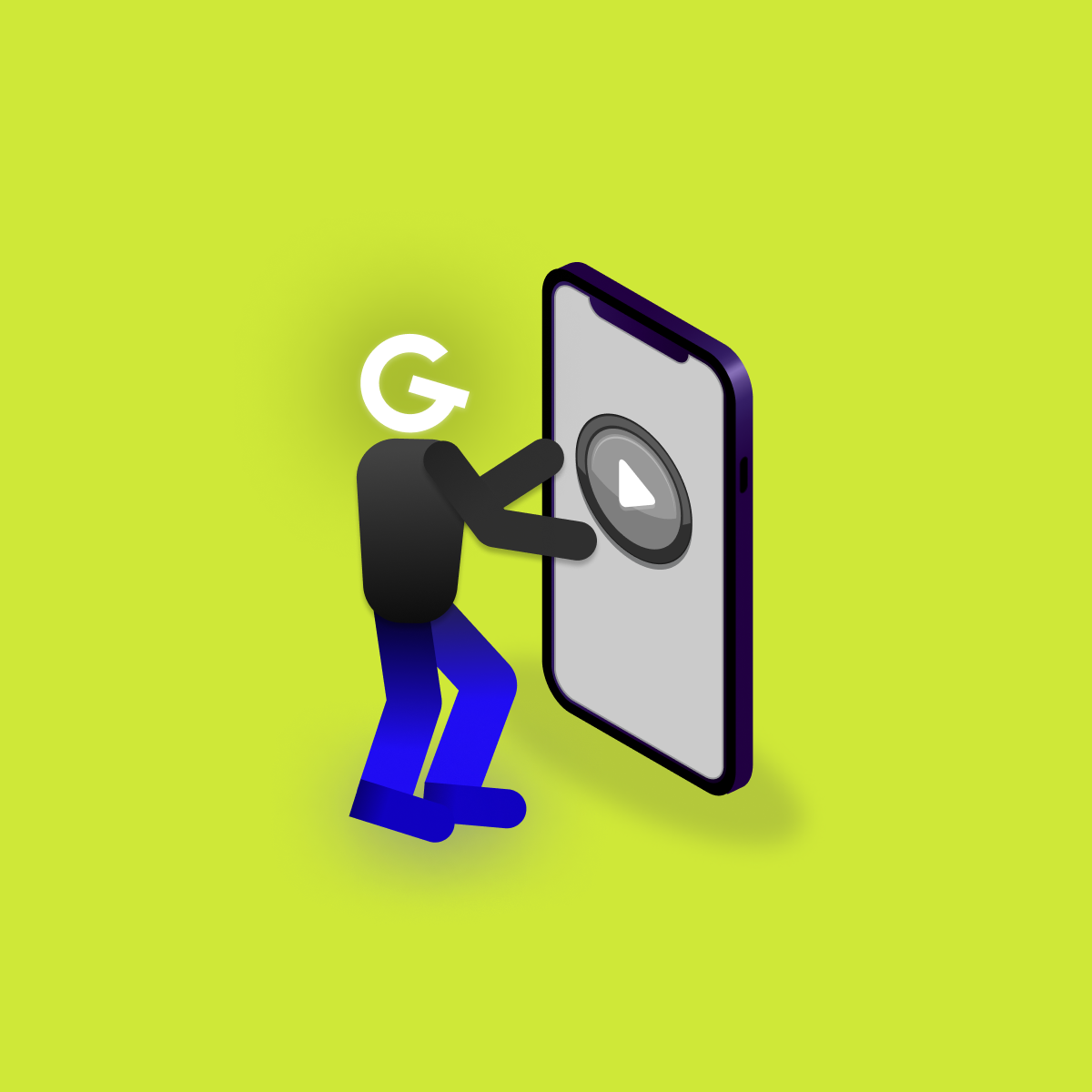
Embedded games in mobile apps: Make your app more attractive

20. Apr 2022
AndroidEvery android developer who writes UI needs to see the results of their efforts at some point, whether for check, debugging or polishing code. One of the options available in Jetpack Compose is to use compose preview built into the Android studio (other options can be building app into device, layout inspector etc.).
To use previews just create a composable functions annotated with @Preview. When composable function is annotated with @Preview, preview should be rendered in design window of Android Studio (for now, integration in AS is not perfect, but developers are still working on improvements. Sometimes multiple builds are needed for preview to render).
Preview annotation provides many ways in which preview can be configured and thus increase productivity (e.g. productivity of people who join the project later). Below, there is minimal @Preview example without use of any of it’s parameters. Function can be written manually or by using prev live template.
@Preview
@Composable
fun ExamplePreview() {
Button(
modifier = Modifier.fillMaxWidth(),
onClick = {},
content = { Text(text = "Button") })
}
Preview annotation located in ui.tooling package has many parameters defining the way how will be composable rendered.
val name: String = "",
val group: String = "",
@IntRange(from = 1) val apiLevel: Int = -1,
val widthDp: Int = -1,
val heightDp: Int = -1,
val locale: String = "",
@FloatRange(from = 0.01) val fontScale: Float = 1f,
val showSystemUi: Boolean = false,
val showBackground: Boolean = false,
val backgroundColor: Long = 0,
@UiMode val uiMode: Int = 0,
@Device val device: String = Devices.DEFAULT
Every preview can be recognized by it’s name, which is name of the preview function by default. In example above, name of preview is ExamplePreview.
@Preview(name = "Some preview name")
@Composable
fun ExamplePreview() {
...
}
Similar previews can be grouped together for better readability. It can be really useful if there is a lot of composable previews.
@Preview(group = "Buttons")
@Composable
fun ButtonEnabledPreview() {...}
@Preview(group = "Buttons")
@Composable
fun ButtonDisabledPreview() {...}
@Preview(group = "Texts")
@Composable
fun TextBigPreview() {...}
@Preview(group = "Texts")
@Composable
fun TextSmallPreview() {...}
Api level used while rendering preview.
By default, the preview window has a width and height of used composable (it has behaviour similar to wrap_content in xml layouts). This can be changed using widthDp and heightDp. Max value for both width and height is 2000. Setting a higher number will not increase the preview width.
@Preview(widthDp = 100, heightDp = 100)
@Composable
fun ExamplePreview() {...}
Determines which locale should be used to render preview (parameter accepts this locale format). If it is not used, default resources are used. This parameter can be useful if your application is multilingual.
In the example below, there is a few things to look at. Multiple preview annotations can be used on single composable, which results in multiple previews. Secondly, second preview uses French resources.
@Preview
@Preview(locale = "fr")
@Composable
fun ButtonPreview() {
Button(
...
content = { Text(text = stringResource(id = R.string.day_monday)) })
}
Can be used to test different font scaling factors.
Boolean which determines if there should be status bar and navigation bar in the preview.
If showBackground boolean is true, default background color will be used behind preview. This color can be customized by backgroundColor parameter. The ARGB color int should be used.
@Preview(showBackground = true, backgroundColor = 0xff0000)
@Composable
fun ExamplePreview() {...}
UI mode configuration used if certain conditions of UI need to be previewed. This is most useful if you want to look at composable in Light or Dark mode (or both).
@Preview(uiMode = UI_MODE_NIGHT_NO)
@Preview(uiMode = UI_MODE_NIGHT_YES)
@Composable
fun ExamplePreview() {...}
Device to use in the preview. There is a list of usable constants in Devices class (mostly Nexus and Pixel devices).
@Preview(device = Devices.PIXEL_3A)
@Composable
fun ExamplePreview() {...}
Interactive mode can be turned on by button above preview. It allows you to interact with preview similarly as on device. It’s simple and quick way to test elements with multiple states like checking a checkbox or using a switch. Interactive mode runs directly inside AS which results in limitations like disabled network access or disabled file access.