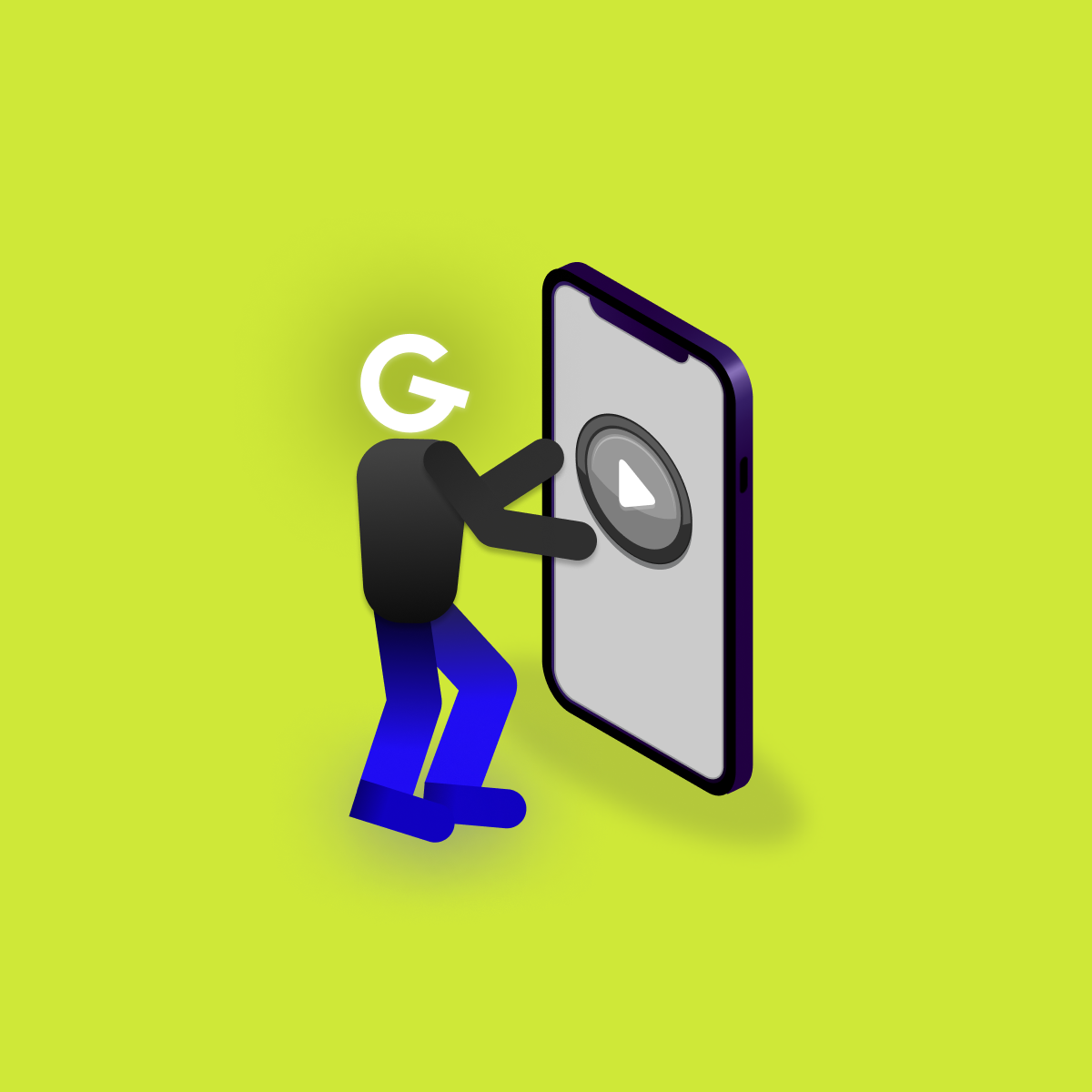
Embedded games in mobile apps: Make your app more attractive

18. Jun 2021
AndroidJava has brought us a lot of joy (and sadness) when we're developing Android applications. It is recognized worldwide, not only in the field of mobile devices. Kotlin is here for a short time, but it has a lot to offer. Read the following comparison from my professional background and form your own opinion.
Java is a well-known object-oriented programming language. The language is designed to have as few implementation dependencies as possible. Java was released in 1996 by Sun Microsystems. Most Java source code is publicly available on GitHub. A huge part of android mobile applications is programmed in Java.
Kotlin 1.0 was released in early 2016 by JetBrains. Kotlin is a static typed programming language. When compiled, it is translated into Java bytecode, which runs on the JVM (Java Virtual Machine). Kotlin is not as popular or widespread as Java, but there are several well-known companies that use this language: Pinterest, Trello, Kickstarter, GoodRequest...
Kotlin was designed from the beginning to be compatible with Java. This perfectly facilitates developers to migrate projects to Kotlin. The language is designed so that we can do more in less time.
class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
class Person(val name: String, val age: Int)
//alebo
data class Person(val name: String, val age: Int)
Kotlin's getters and setters are already automatically generated for us. Of course, let's not forget to mention the famous copy function. Data classes are fixed, which makes it easier for us to work in multiple thread programs. Copy copies an instance to us and creates a new one where we can move other values.
val person = Person("Android developer", 30)
val copyPerson = person.copy(age = 50)
Nullable is a big disadvantage in Java. When we define the String name variable, we cannot say with certainty that whether or not name has a value in it. It can usually happen that when working with a variable, we can get a NullPointerException.
In Kotlin we have the choice to define the variable as Nullable (in Kotline it is called Optional) val name: String? . Here we can safely say that a variable can contain null. If we define without a question mark, we can say with certainty that a variable can never be null.
Person person = new Person(null, 21);
try {
System.out.println(person.getName().charAt(0));
} catch (NullPointerException e) {
System.out.println("No name");
}
data class Person(val name: String?, val age: Int)
val person = Person(null, 30)
person.name?.get(0)?.let(::println)
In Java we have to take into account that it can be null, in Kotlin we can ensure this with the character `?` Which will ensure that the instruction does not continue in the case of null.
Speed
We can say that Java and Kotlin have the same "coding" speed. Kotlin has more concise designs that allow the developer to write less. But it has one catch, finding a solution to a problem in Kotlin takes more time than in Java. This means that Kotlin has a greater cognitive load than Java. If you are a fantastic abstract thinker, Kotlin is your choice.
In terms of compilation speed, Kotlin is a bit slower than Java. In both cases, the instructions are translated into a Java bytecode. Kotlin has more features than Java, so we can see a difference. Also, the execution of instructions is faster in Java. However, I must point out that the difference is minimal and unobservable with today's machines.
Stability
Java has been with us for decades. Java versions have long-term support since Java 8, anything that would go wrong, the founders can fix it quickly. Kotlin is only here for a short time and is still evolving. There is still the possibility that some features of the language that are supported today in newer versions will no longer be, which can cause problems with updates.
Because Java has been with us for a long time, there are many books and instructions or documentation from which we can draw knowledge and know how. Kotlin has some good official sources, but that's about it.
Kotlin & Android
Kotlin works with Java bytecode, there is no problem. If we look at Google trends, Java is still much more popular in Slovakia than Kotlin. However, Kotlin is currently designed for the development of mobile applications. JetBrains are not only its authors, but also the authors of the most famous Android Studio interface. Learning Kotlin can be more challenging because it requires more cognitive thinking. In Java, we write more code to solve the problem, but we have more know-how and ready-made solutions from which we can draw. It is no problem to program the android application purely in Kotlin. It will run as well as in Java.
trends.embed.renderExploreWidget("TIMESERIES", {"comparisonItem":[{"keyword":"/m/07sbkfb","geo":"SK","time":"today 12-m"},{"keyword":"/m/0_lcrx4","geo":"SK","time":"today 12-m"}],"category":0,"property":""}, {"exploreQuery":"geo=SK&q=%2Fm%2F07sbkfb,%2Fm%2F0_lcrx4&date=today 12-m,today 12-m","guestPath":"https://trends.google.com:443/trends/embed/"});
Kotlin or Java?
Personally, I prefer Kotlin over Java. The fact that less code does more is a big factor for me. Beauty is in simplicity. If I read the code, I spend less time searching for Kotlin than I do for Java. Understanding the basics and hidden tricks of the language has been difficult, and over the years there are still features and solutions that I come up with gradually. Static extensions (Kotlin extensions) of existing classes is also a big plus.
As far as working with multiple threads is concerned, we have native support for Kotlin Coroutines in Kotlin. In Java, we have to take RxJava to help or use callbacks. Coroutines again make the code easier to read, but it requires an even more abstract imagination. Again, in the opposite case of Java, with RxJava it is necessary to study longer code and documentation.
Are you interested in this topic? I have prepared an introduction to Kotlin Coroutines as well.
What should I start with?
This decision is up to you. I think getting started with Java is easier. It is more widespread, there are many more manuals and handbooks. Then I would bridge to Kotlin. With Java, you will understand the wheels of the program faster and what is happening where. It will be easier to learn then Kotlin. It should also be taken into account that Java is a multiplatform language, which is not only extended to the development of mobile applications, but also to other devices, servers or operating systems.
Programming in Kotlin is fun, programming in Java is a job.
If you prefer simplicity and straightforwardness, definitely Java.
If you like challenge and abstractness, definitely Kotlin.
Don't forget to visit our useful #goodroidtips