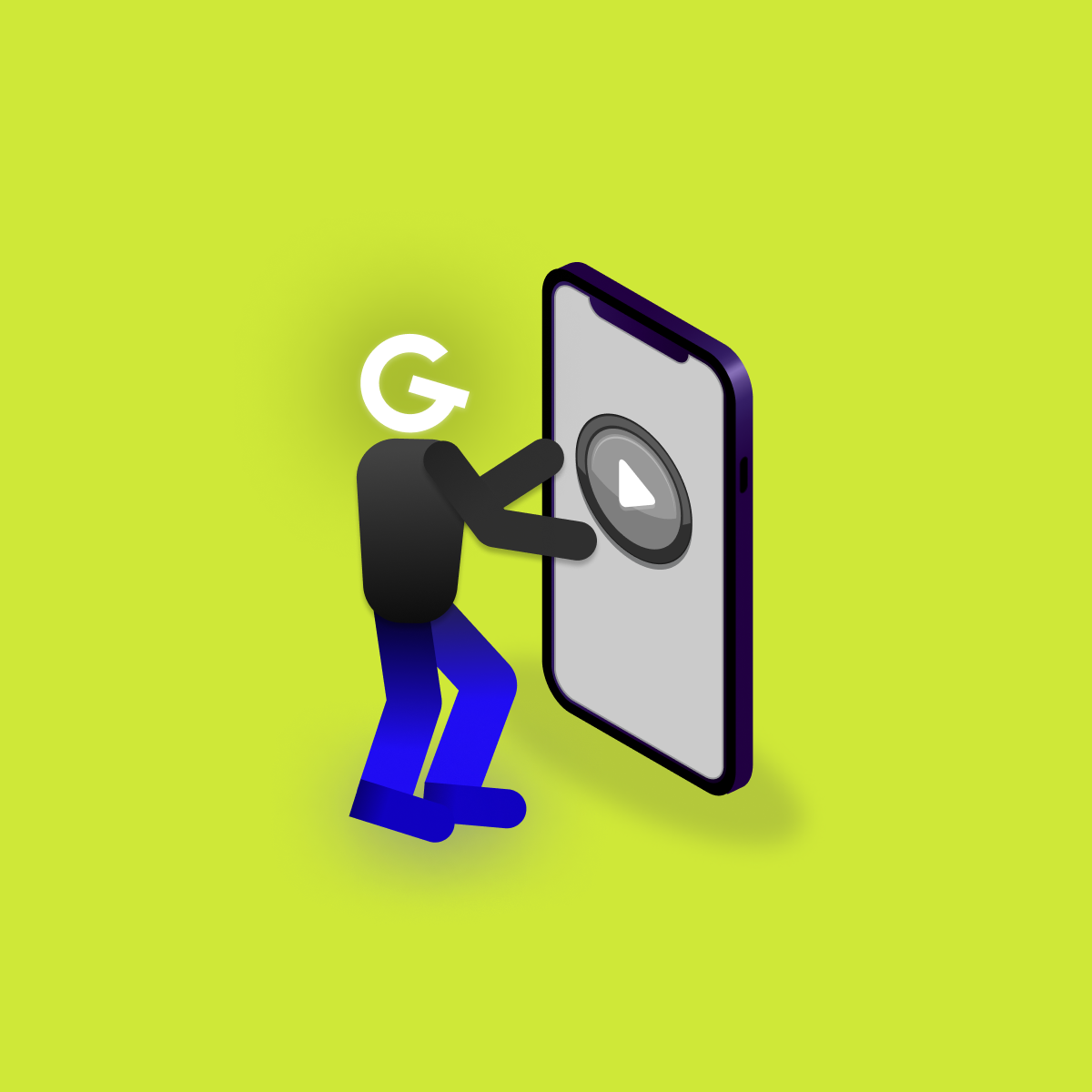
Embedded games in mobile apps: Make your app more attractive

16. Aug 2021
AndroidFor our GoodRequest Android team, the most important aspect of programming is the readability. We want our code to be readable because it affects all the main goals of our company. You can’t create apps that are error prone, easy to maintain, easy to modify, etc. if your code is messy and hard to understand.
When we develop Android apps, we prefer readability over ease of writing. There are a lot of things that affect code readability like names of variables and functions, language syntax, use of pure functions, etc. But readability can be also increased by good code alignment. And this doesn’t only mean how many tabs or spaces you use. Aligning the same parts of code in columns can help you see what is different. Or it can help you ignore what is the same.
For example when you are creating data classes with more fields. It is more readable when you put field names in one column and data types in another.
data class EmployeeDetail(
val employee: Employee,
val personalNumber: PersonalNumber,
val hasCard: Boolean,
val hasPin: Boolean,
val fingerprints: List<Finger>
)
data class EmployeeDetail(
val employee : Employee,
val personalNumber : PersonalNumber,
val hasCard : Boolean,
val hasPin : Boolean,
val fingerprints : List<Finger>
)
Or if you declare a function with more parameters. It is easier to read parameter names and their data types.
fun View.actionsPage(
actions: List<Action>,
grid: Grid,
itemsPerPage: Int,
position: Int,
settings: Settings
) = whenAttached { }
fun View.actionsPage(
actions : List<Action>,
grid : Grid,
itemsPerPage : Int,
position : Int,
settings : Settings
) = whenAttached { }
Another example is something repetitive as setting string values to TextViews.
date.text = string(R.string.attendance_detail_header_date)
category.text = string(R.string.attendance_detail_header_category)
time.text = string(R.string.attendance_detail_header_time)
duration.text = string(R.string.attendance_detail_header_duration)
shift.text = string(R.string.attendance_detail_header_time)
arrival.text = string(R.string.attendance_detail_header_arrival)
If you align the same code in columns you can easily see the mistake now (shift TextView is set with wrong string :) ), because you can visually ignore the same parts and focus on what is important.
date .text = string(R.string.attendance_detail_header_date)
category.text = string(R.string.attendance_detail_header_category)
time .text = string(R.string.attendance_detail_header_time)
duration.text = string(R.string.attendance_detail_header_duration)
shift .text = string(R.string.attendance_detail_header_time)
arrival .text = string(R.string.attendance_detail_header_arrival)
We use code alignment like this a lot and it can be quite time consuming. So we decided to create a plugin for Android Studio called GoodFormatter.
It’s limited to 50 lines of code because it’s quite heavy to process long strings and align them. We are working on version 2.0 which will use PSI tree data structure and therefore allow us to optimize the alignment process.
But there are drawbacks too. So to sum things up let’s look at pros and cons.