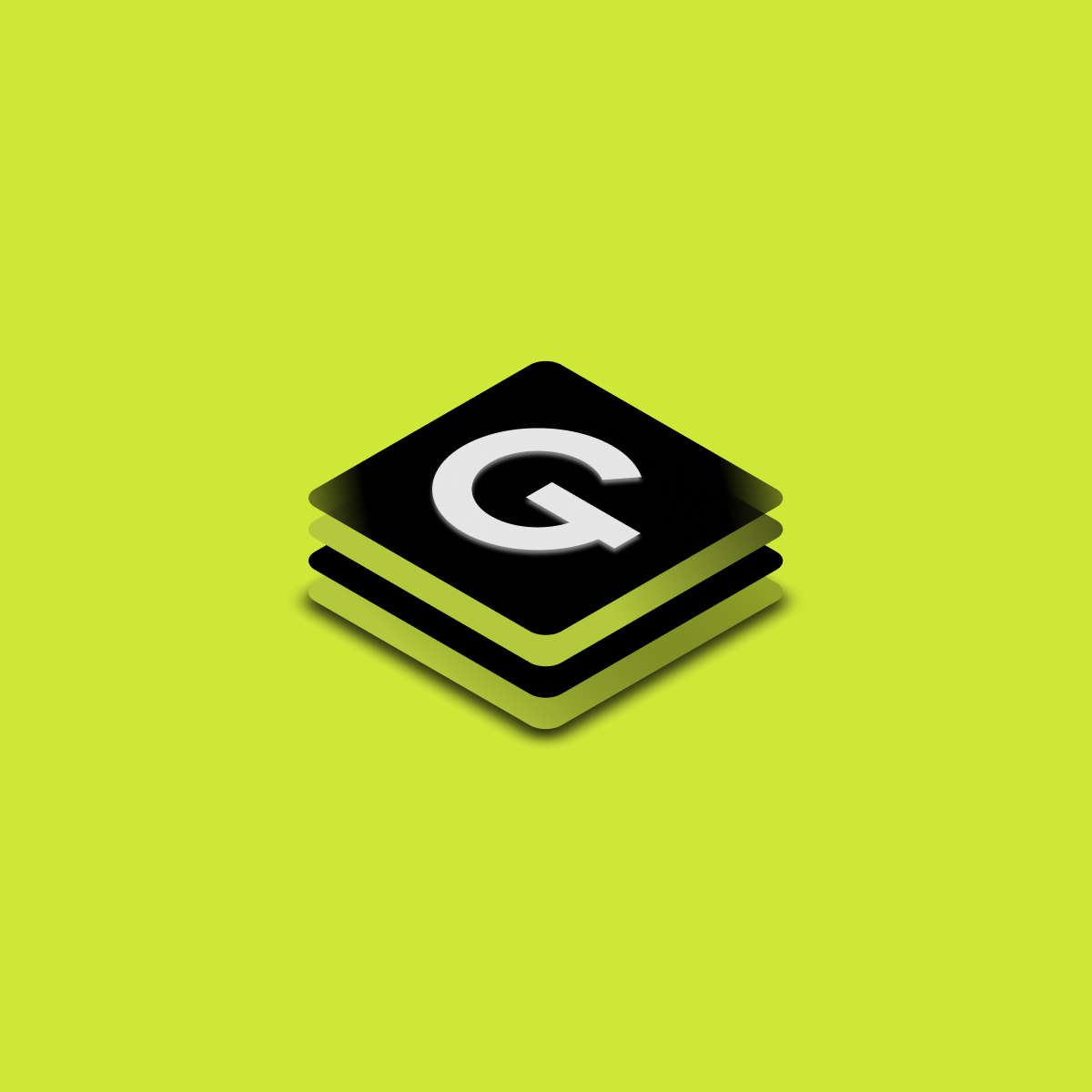
Our Frontend Tech Stack: Focused On Performance And Scalability

6. Jun 2022
FrontendIn the article, we will deal with the issue of "drag and drop", which everyone has met in practice. Many of you always reach for a library when implementing this feature, which is of course "easier". But this may not be the case every time. Libraries are mostly dependent on other modules that you do not need, or you still need to modify the library to meet your functionality requirements.
Let's take a look at the native capabilities of the HTML5 drag and drop API itself.
Using the native HTML5 interface, we can enable virtually any HTML element. It's easy, just set the draggable = true attribute for this element.
<div
className={'drag-item-list'}
key={item.label}
draggable={true}
>
<DragOutlined /> {item.label}
</div>
By assigning this attribute, it is possible to activate the drag and drop function, for example for the following elements: image, file, link ...
Next, we will show what callbacks the interface provides us in case of a change in the position of the moved element.
The native interface provides us with a number of different events that we can use to monitor and manage the entire drag and drop process. Using these events, it is possible to perform the necessary operations on the displayed data with which the user interacts. Below we will mention selected ones, without which it is not possible to use this functionality.
event dragStart → onDragStart
This event handler is used to process the element that is currently being moved and in the example it is used to send data.
The dataTransfer.setData () function sets the data type and value of the moved data.
onDragStart={(event) => {
event.dataTransfer.setData('text', JSON.stringify({ label: 'test', value: '1' }))
}}
In this example, the data type is a text and a value object from the label and value attributes.
The onDrop event handler provides the ability to retrieve data about a moved element in the active drop zone. As an example, we can mention a simple list in which there would be different types of activities and the user would be able to move these elements to the drop zone, where it would then be possible to change the order of activities from the list.
const onDrop = (event: any) => {
try {
// parse droped element data
const data = JSON.parse(event.dataTransfer.getData('text'))
addElement(data)
} catch (error) {
console.log(error)
}
}
The example shows the onDrop function, which ensures the acquisition and processing of data of an element inserted in the list of activities. To get the data, you need to call the dataTransfer.getData ('text') method. Since in our case it is an object, we prepare it into JSON format and call the function to add the activity to the list.
event dragOver → onDragOver
It is used to process the event if the moved element "passes" through the drop zone. If the user moves the element through the active drop zone, it is necessary to prevent the element from being added to the drop zone, which is the default behavior, by calling the preventDefault () and stopPropagation () methods.
onDragOver={(event) => {
event.preventDefault()
event.stopPropagation()
}}
event dragLeave → onDragLeave
We can use this event handler to remove an element that the user is currently moving, but has not yet "released" it into the target drop zone / element, or released the element outside the target zone. As an example, I will move the activity between the individual columns, where an animation of the removal of the moved element or an animation above the drop zone is required.
event dragEnter → onDragEnter
The handler monitors the element that the user is moving and that has entered the drop zone / element boundary. The usage has the opposite of onDragLeave, ie in case the moved element entered the drop zone, but the move was not terminated by a drop action. Then we can add the element.
The event is triggered if the element moved by the user has been "placed" in the drop zone. The usage is similar to the onDragLeave event.
As an example, I chose a simple sheet of elements, which will be located on the left and a drop zone will be located on the right, where the user will be allowed to add individual elements from the sheet.
I believe that I have introduced and clarified the possibilities of using the native HTML API interface for drag and drop. If you again encounter the requirement to implement this functionality in your project, do not forget to consider all the advantages and disadvantages of using a library that provides these options or take the solution from this article 🙂.