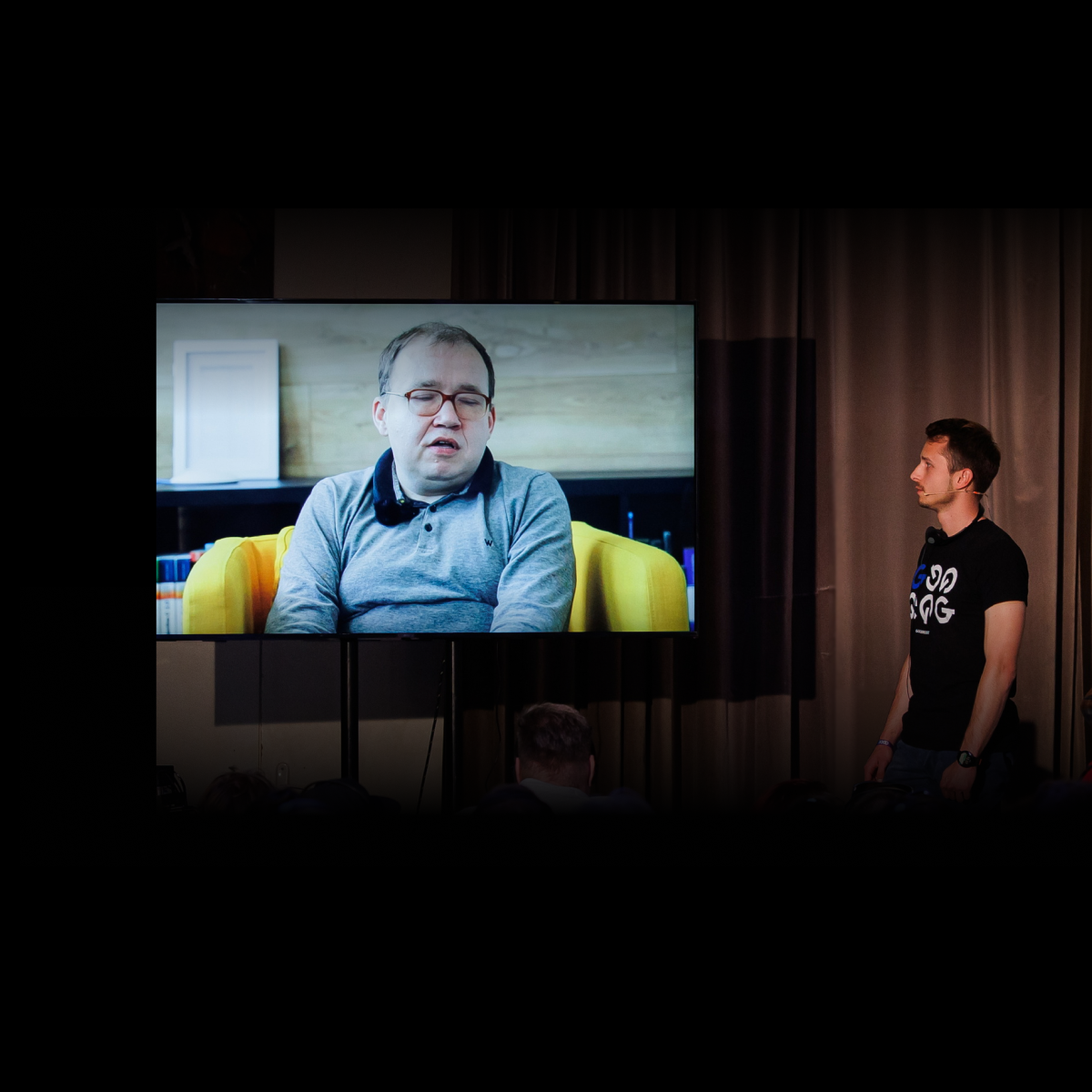
Webinár: Ako ľudia so zdravotným postihnutím používajú mobilné aplikácie a ako ich testovať

23. Mar 2021
AndroidConstraint layout je typom ViewGroup, ktorý umožňuje vytvárať zložité rozloženia s hierarchiou plochých view. Plná sila CL prichádza od jeho helpers.
Najjednoduchšie z ConstraintLayout helpers. Kontroluje viditeľnosť viacerých (alebo jedného) odkazovaných views. Má tendenciu byť užitočný, ak chcete nastaviť viac views do stavu VISIBLE alebo GONE, ale nechcete ich zabaliť do wrapper ViewGroup, aby vaša štruktúra XML mohla zostať nezmenená.
Na odkazovanie na views vo vnútri skupiny možno použiť atribút constraint_referenced_ids.
app:constraint_referenced_ids="text,button"
V nasledujúcom príklade je možné ovládať viditeľnosť textu a tlačidiel nastavením viditeľnosti na Group Helper. Napr.
//Doing this somewhere in code shows text and button
group.visibility = View.VISIBLE
//Doing this somewhere in code hides text and button
group.visibility = View.GONE
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.constraintlayout.widget.Group
android:id="@+id/group"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:constraint_referenced_ids="text,button"/>
<com.google.android.material.textview.MaterialTextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Android Text"
app:layout_constraintTop_toTopOf="parent"/>
<com.google.android.material.button.MaterialButton
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Android"
app:layout_constraintTop_toBottomOf="@id/text"/>
</androidx.constraintlayout.widget.ConstraintLayout>
V prípade, že sa na jeden view odkazuje z viacerých skupín, declaration order XML definuje konečnú viditeľnosť (posledná skupina v XML má posledné slovo).
Prakticky to isté ako Group helper, ale sú podporovanézmeny prekladu, mierky a rotácie.
Napr. rotácia viacerých views môže vyzerať takto.
image1.setOnClickListener { layer.rotation = Random.nextInt(60).toFloat()}
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/image1"
android:layout_width="64dp"
android:layout_height="64dp"
android:src="@drawable/background_badge_green"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toStartOf="@id/image2"/>
<ImageView
android:id="@+id/image2"
android:layout_width="64dp"
android:layout_height="64dp"
android:src="@drawable/background_badge_red"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toEndOf="@id/image1"
app:layout_constraintEnd_toEndOf="parent"/>
<androidx.constraintlayout.helper.widget.Layer
android:id="@+id/layer"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:constraint_referenced_ids="image1, image2"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Neviditeľný helper object pre Constraint Layout (na zariadenísa nikdy nezobrazí).
Hlavným účelom guideline je umiestniť child of ConstraintLayout na určité konkrétne miesto (napr. 100 dpi od začiatku obrazovky alebo 25% od dolnej časti obrazovky ...) Guideline môže byť buď vertikálna (nulová šírka, výška nadradeného rozloženia obmedzenia) alebo horizontálna (šírka rozloženia obmedzenia rodiča, nulová výška).
Na definovanie orientácie guideline môžete použiť atribút orientácie.
android:orientation="vertical"
OR
android:orientation="horizontal"
Najdôležitejšou vlastnosťou guideline je jeho poloha. Guideline je možné umiestniť tromi rôznymi spôsobmi pomocou atribútov layout_constraintGuide_begin, layout_constraintGuide_end a layout_constraintGuide_percent. Ovplyvňuje ich aj orientácia.
//100dp from start
android:orientation="vertical"
app:layout_constraintGuide_begin="100dp"
OR
//100dp from bottom
android:orientation="horizontal"
app:layout_constraintGuide_end="100dp"
OR
//14% from top
android:orientation="horizontal"
app:layout_constraintGuide_percent="0.14"
Akékoľvek direct child of Constraint Layout môže byť obmedzené na guideline, ale samotná guideline nemôže mať žiadne obmedzenia. Pozíciu guidelines určujú iba uvedené atribúty. Tu je príklad tlačidla umiestneného na 25% od hornej časti obrazovky.
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
app:layout_constraintGuide_percent="0.25" />
<com.google.android.material.button.MaterialButton
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Android"
app:layout_constraintTop_toTopOf="@id/guideline"
app:layout_constraintBottom_toBottomOf="@id/guideline"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout
Barrier helper je podobná ako v Guideline. Hlavný rozdiel medzi nimi je v tom, že Barrier je flexibilná. V atribúte constraint_referenced_ids zaberá viac views a vytvára virtual guideline založené na najextrémnejšom pohľade z referenčných views (najextrémnejšie view je najširšie alebo najvyššie view). Ak sa počas behu zmení šírka (alebo výška) najextrémnejšieho pohľadu, barrier sa automaticky posunie podľa nej. Každý Barrier musí mať atribút BarrierDirection.
app:barrierDirection="start" //Barrier is positioned at the start of referenced views
app:barrierDirection="end" //Barrier is positioned at the end referenced views
app:barrierDirection="top" //Barrier is positioned above referenced views
app:barrierDirection="bottom" //Barrier is positioned below referenced views
Barrier býva užitočná, ak chcete obmedziť pohľad pod (alebo nad, na konci, na začiatku) iných pohľadov. Napr. usecase, keď tlačidlo musí byť pod textViews, bez ohľadu na to, aké sú dlhé. Mali by ste si všimnúť,že môžete obmedziť pohľady na Barrier (na rozdiel od guideline).
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.constraintlayout.widget.Barrier
android:id="@+id/barrier"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:barrierDirection="bottom"
app:constraint_referenced_ids="text1,text2" />
<com.google.android.material.textview.MaterialTextView
android:id="@+id/text1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Android Text"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toStartOf="@id/text2"/>
<com.google.android.material.textview.MaterialTextView
android:id="@+id/text2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Android Text slightly wider Android Text slightly wider"
app:layout_constraintStart_toEndOf="@id/text1"
app:layout_constraintEnd_toEndOf="parent"/>
<com.google.android.material.button.MaterialButton
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Android"
android:layout_marginTop="16dp"
app:layout_constraintTop_toBottomOf="@id/barrier"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Páčil sa Vám tento článok? Nezabudnite si prečítať aj druhú časť o ConstraintLayout helpers a sledovať ma na LinkedIn a dozviete sa viac :)