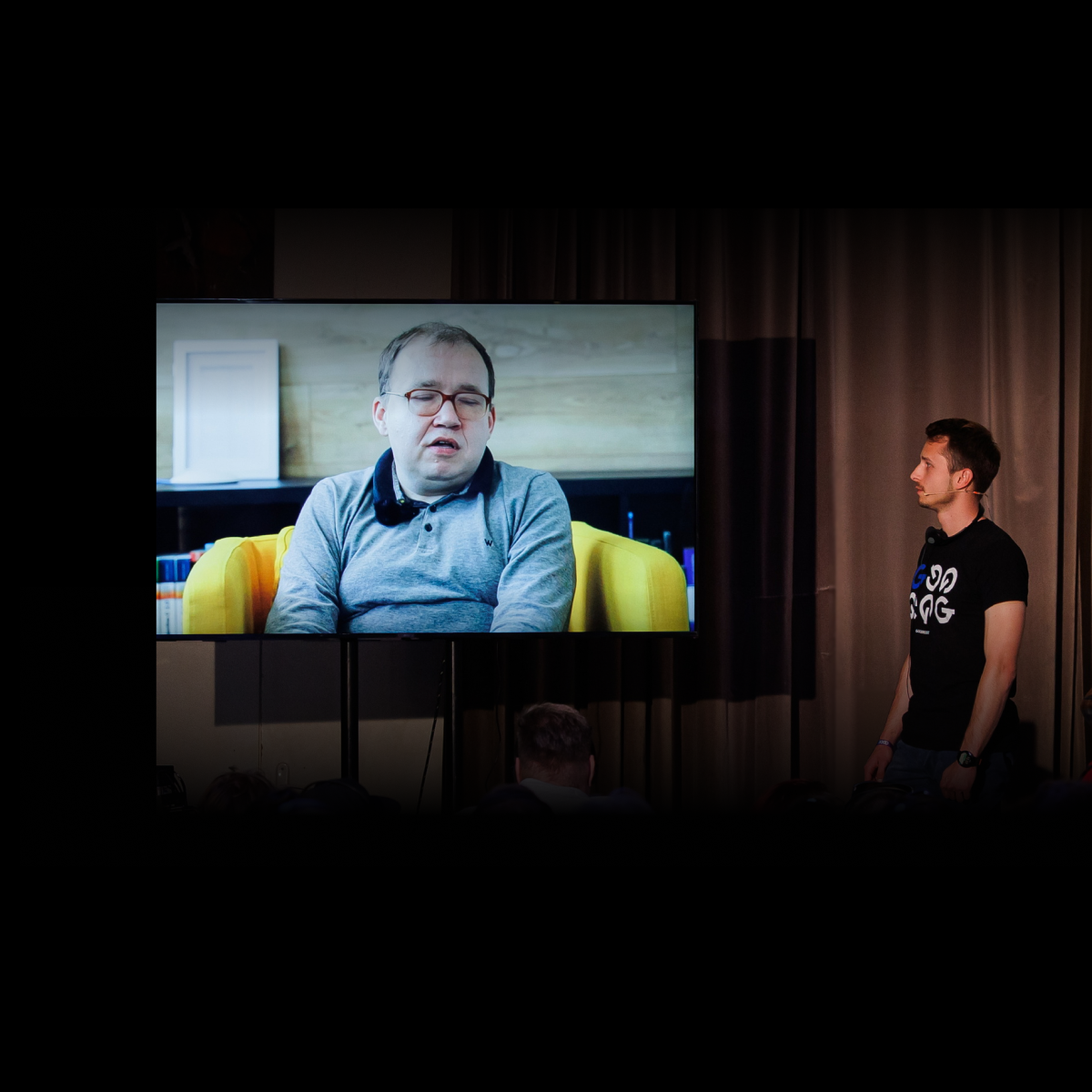
Webinár: Ako ľudia so zdravotným postihnutím používajú mobilné aplikácie a ako ich testovať

19. Apr 2021
AndroidPrinášam vám druhú časť článku o Constraint Layout Helpers, čo sú typy ViewGroup, ktoré vám umožňujú vytvárať zložité rozloženia. Plná sila Constraint Layouts pochádza od jeho helpers, takže som o nich pripravil článok.
V prvej časti úvodu k Constraint Layout Helpers som predstavil Group, Guidline, Layer a Barrier. Ak ste ho ešte nevideli, nezabudnite si prečítať prvý diel!
Placeholder je helper, ktorý pomáha pri umiestňovaní ďalších pohľadov. Keď zavoláte metódu setContentId () na placeholder objekt niekde v kóde, placeholders sa stanú content view. Ak content view už na obrazovke existuje, bude sa s ním po volaní setContentId () zaobchádzať ako s pôvodným umiestnením.
Napr. keď kliknete na niektoré z horných kruhov, zavolá sa placeholder.setContentId (), zástupný symbol sa stane "clicked" pohľadom (kliknutý pohľad sa presunie do polohy zástupcu, zdedí obmedzenia placeholderu).
val onClickListener = View.OnClickListener { view ->
TransitionManager.beginDelayedTransition(content) //For animation effect
placeholder.setContentId(view.id) //Set view to placeholder
}
image1.setOnClickListener(onClickListener)
image2.setOnClickListener(onClickListener)
image3.setOnClickListener(onClickListener)
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/content"
android:paddingTop="16dp">
<ImageView
android:id="@+id/image1"
android:layout_width="64dp"
android:layout_height="64dp"
android:src="@drawable/background_badge_green"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toStartOf="@id/image2"/>
<ImageView
android:id="@+id/image2"
android:layout_width="64dp"
android:layout_height="64dp"
android:src="@drawable/background_badge_red"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toEndOf="@id/image1"
app:layout_constraintEnd_toStartOf="@id/image3"/>
<ImageView
android:id="@+id/image3"
android:layout_width="64dp"
android:layout_height="64dp"
android:src="@drawable/background_badge_black"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toEndOf="@id/image2"
app:layout_constraintEnd_toEndOf="parent"/>
<androidx.constraintlayout.widget.Placeholder
android:id="@+id/placeholder"
android:layout_width="64dp"
android:layout_height="64dp"
android:layout_marginBottom="16dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Nenechajte si ujsť moje #goodroidtips I. alebo II. , články o užitočných tipoch a trikoch pre Android developerov.
Podobne ako v prípade Group, Flow prevezme ID zobrazení do atribútu constraint_referenced_ids a automaticky medzi nimi vytvorí reťazové správanie. Reťazové správanie (spôsob, akým pohľady pretekajú Flow) je určené atribútom wrapMode v Flow.
//Default behavior of Flow, just creates chain between referenced views
//If views do not fit into Flow, they will overflow the Flow
app:flow_wrapMode="none"
OR
//If views do not fit into Flow, they jump on another line and take place evenly
app:flow_wrapMode="chain"
OR
//If views do not fit into Flow, they jump on another line and align to create rows and columns
app:flow_wrapMode="aligned"
Ako sme už povedali, Flow automaticky vytvára reťaz medzi svojimi prvkami. Štýl reťazca je možné nakonfigurovať pomocou atribútu flow_horizontalStyle alebo flow_verticalStyle na základe orientácie Flow. Správanie sa chain style je v zásade rovnaké ako pri chains.
For horizontal Flow
app:flow_horizontalStyle="spread" //Default
OR
app:flow_horizontalStyle="packed"
OR
app:flow_horizontalStyle="spread_inside"
Prvý a posledný reťazec v Flow môže mať rôzne atribúty štýlu ako iné, napr. pre prvý reťazec definujeme flow_firstHorizontalStyle = "spread_inside" pre posledný reťazec definujeme flow_lastHorizontalStyle = "packed". Všetky ostatné reťazce v strede prijali predvolené flow_horizontalStyle = "spread", pretože sme nič nedefinovali.
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.constraintlayout.helper.widget.Flow
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
app:flow_wrapMode="chain"
app:flow_maxElementsWrap="2"
app:flow_firstHorizontalStyle="spread_inside"
app:flow_lastHorizontalStyle="packed"
app:constraint_referenced_ids="circle1, ..., circle9"/>
//Include 9 circles
<include
android:id="@+id/circle1"
layout="@layout/circle"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Flow je možné prispôsobiť pomocou mnohých ďalších atribútov, napr.
//Orientation of Flow, can be vertical or horizontal
android:orientation="vertical"
//Maximum number of elements in one row or column
app:flow_maxElementsWrap="3"
//Vertical or horizontal gap between referenced views
app:flow_horizontalGap="20dp"
app:flow_verticalGap="20dp"
Atribúty circular positioning vám umožňujú obmedziť view na iný view v určenom uhle a vzdialenosti. Dôležitými atribútmi, keď chceme kruhovo umiestniť pohľady, sú
app:layout_constraintCircle="@id/baseImage" //Constrained base view
app:layout_constraintCircleRadius="90dp" //Radius of the circle
app:layout_constraintCircleAngle="45" //Degree of the circle on which we display the view
Napr. umiestnenie obrázkov 1, 2 a 3 okolo baseImage v 0, 45 a 90 stupňoch.
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/image1"
android:layout_width="32dp"
android:layout_height="32dp"
android:src="@drawable/background_badge_green"
app:layout_constraintCircle="@id/baseImage"
app:layout_constraintCircleRadius="90dp"
app:layout_constraintCircleAngle="0"/>
<ImageView
android:id="@+id/image2"
android:layout_width="32dp"
android:layout_height="32dp"
android:src="@drawable/background_badge_green"
app:layout_constraintCircle="@id/baseImage"
app:layout_constraintCircleRadius="90dp"
app:layout_constraintCircleAngle="45"/>
<ImageView
android:id="@+id/image3"
android:layout_width="32dp"
android:layout_height="32dp"
android:src="@drawable/background_badge_green"
app:layout_constraintCircle="@id/baseImage"
app:layout_constraintCircleRadius="90dp"
app:layout_constraintCircleAngle="90"/>
<ImageView
android:id="@+id/baseImage"
android:layout_width="64dp"
android:layout_height="64dp"
android:layout_marginBottom="16dp"
android:layout_marginStart="16dp"
android:src="@drawable/background_badge_black"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>